图书介绍
Java基础教程 英文PDF|Epub|txt|kindle电子书版本网盘下载
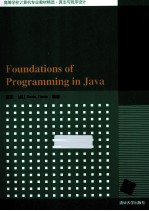
- 董东,(澳)芬尼编著 著
- 出版社: 北京:清华大学出版社
- ISBN:9787302325512
- 出版时间:2013
- 标注页数:408页
- 文件大小:73MB
- 文件页数:420页
- 主题词:JAVA语言-程序设计-高等学校-教材-英文
PDF下载
下载说明
Java基础教程 英文PDF格式电子书版下载
下载的文件为RAR压缩包。需要使用解压软件进行解压得到PDF格式图书。建议使用BT下载工具Free Download Manager进行下载,简称FDM(免费,没有广告,支持多平台)。本站资源全部打包为BT种子。所以需要使用专业的BT下载软件进行下载。如BitComet qBittorrent uTorrent等BT下载工具。迅雷目前由于本站不是热门资源。不推荐使用!后期资源热门了。安装了迅雷也可以迅雷进行下载!
(文件页数 要大于 标注页数,上中下等多册电子书除外)
注意:本站所有压缩包均有解压码: 点击下载压缩包解压工具
图书目录
Chapter 1 Introduction to Java Programming1
1.1 Abstraction1
1.2 Development Environment and Running Environment3
1.3 Programming in the Command Prompt Window4
1.4 Programming in Eclipse7
1.5 Java Application Structure11
1.6 Code Style12
1.6.1 Naming Conventions13
1.6.2 Indentation and Spacing13
1.6.3 Block Styles14
1.7 Comments15
1.8 Java and Development Tools17
1.9 Foundations of Object-Oriented Programming17
1.10 New Terminology19
1.11 NewWords and Expressions21
1.12 Hands on Lab21
1.12.1 Installing JDK and Eclipse IDE21
1.12.2 Programming in Command Prompt Window22
1.12.3 Programming in Eclipse24
1.12.4 Exporting and Importing Java Projects in Eclipse28
1.13 Exercises32
Chapter 2 The Basics of Java Language33
2.1 Identifiers33
2.2 Primitive Data Types34
2.3 Literals36
2.4 Variables37
2.5 Operators40
2.5.1 Assignment40
2.5.2 Arithmetic Operators41
2.5.3 Relational Operators41
2.5.4 Logical Operators42
2.5.5 Bitwise Operators43
2.5.6 Conditional Operator43
2.5.7 Operator Precedence44
2.6 Expressions and Statements45
2.7 The Scanner Class46
2.8 Control Structures48
2.8.1 Sequence Structures49
2.8.2 Selection Structures49
2.8.3 Repetition Structures53
2.8.4 Branching Statements56
2.9 Arrays57
2.10 Built-in Java Classes61
2.10.1 Java Strings61
2.10.2 The StringBuffer Class66
2.10.3 Random Numbers67
2.10.4 BigInteger Objects68
2.10.5 Date and Time68
2.10.6 Wrapper Classes71
2.11 Command Line Arguments73
2.12 New Terminology74
2.13 New Words and Expressions75
2.14 Hands on Lab76
2.14.1 Getting Input from Keyboard via ScannerClass76
2.14.2 Converting String Type into int Type77
2.14.3 Two-Dimensional Array of int79
2.14.4 Java Strings80
2.15 Exercises81
Chapter 3 Classes and Objects87
3.1 Class Declaration87
3.2 Creating Objects94
3.3 Accessing Objects via Reference Variables94
3.4 Object Reference this95
3.5 Parameter Passing96
3.6 Returning from a Method102
3.7 Method Overloading103
3.8 Class Variables and Instance Variables104
3.9 Class Methods and Instance Methods106
3.10 The Scope of Variables108
3.11 Garbage Collection110
3.12 Reflection110
3.13 Code Organization112
3.13.1 Java API113
3.13.2 Package Customs113
3.14 Pattern Matching with Regular Expressions115
3.15 Summary of Creating and Using Classes and Objects124
3.16 New Terminology124
3.17 New Words and Expressions126
3.18 Hands on Lab126
3.18.1 Static Variables and Instance Variables126
3.18.2 Static Methods and Instance Methods127
3.19 Exercises128
Chapter 4 Inheritance,Interface and Polymorphism136
4.1 The Concept of Inheritance136
4.2 Constructors of Superclass and Subclass140
4.3 Method Overriding142
4.4 Upcasting and Downcasting144
4.5 Abstract Class and Abstract Method146
4.6 Interfaces148
4.7 Polymorphism153
4.8 Final Classes and Final Members154
4.9 Access Control156
4.10 Methods in the Object Class160
4.10.1 The toString Method161
4.10.2 The equals Method161
4.10.3 The hashCode Method163
4.10.4 The clone Method164
4.11 Comparison of Objects169
4.12 New Terminology172
4.13 New Words and Expressions172
4.14 Hands on Lab172
4.14.1 Reuse Class via Inheritance172
4.14.2 Constructor Calling Chain173
4.14.3 Overriding Method174
4.14.4 Runtime Polymorphism175
4.14.5 Interface175
4.14.6 Access Control177
4.15 Exercises177
Chapter 5 Exception Handling186
5.1 Introduction186
5.2 Handling Exceptions189
5.3 The Finally Block192
5.4 User-defined Exceptions195
5.5 Benefits of Java Exception Handling Framework197
5.6 Assertions200
5.7 New Terminology203
5.8 New Words and Expressions203
5.9 Hands on Lab203
5.9.1 Classpath and ClassNotFoundException203
5.9.2 Catch a Runtime Exception206
5.9.3 Create Your Own Exception Class207
5.10 Exercises207
Chapter 6 Collections Framework213
6.1 Introduction213
6.2 Set Interface217
6.3 List Interface222
6.3.1 ArrayList223
6.3.2 Algorithms for List227
6.4 Stack231
6.5 Queue Interface235
6.6 Map Interface236
6.7 Generics240
6.8 New Terminology245
6.9 New Words and Expressions245
6.10 Hands on Lab246
6.10.1 View JDK Source Code in Eclipse246
6.10.2 Using HashSet and TreeSet247
6.10.3 Using List249
6.10.4 Using Map250
6.10.5 Sorting and Searching250
6.11 Exercises251
Chapter 7 Stream I/O256
7.1 Manipulating Disk Files and Folders256
7.2 Streams260
7.2.1 Byte Streams261
7.2.2 Buffered Byte Streams264
7.2.3 Data Streams265
7.2.4 Character Streams269
7.2.5 Buffered Character-based I/O271
7.3 Reading Values ofVarious Types by Scanner273
7.4 Formatted-Text Printing with printf() Method278
7.5 Object Serialization283
7.6 Standard I/O and Redirection285
7.7 Character Sets and Unicode286
7.8 New Terminology288
7.9 New Words and Expressions289
7.10 Hands on Lab289
7.10.1 Buffered Stream289
7.10.2 DataInputStream and Data OutputStream290
7.10.3 Character Stream290
7.10.4 printf() Method293
7.10.5 Object Serialization293
7.10.6 Random Access File293
7.11 Exercises294
Chapter 8 Creating a GUI with JFC/Swing299
8.1 Introduction299
8.2 Using Swing APIs and Layout Managers299
8.3 Swing Components303
8.4 Component Inclusion Relationship in a GUI Application306
8.5 Dialogs308
8.6 Layout Management312
8.7 Menus317
8.8 Basic Components and Their Events320
8.9 Events322
8.10 MVC331
8.11 New Terminology339
8.12 New Words and Expressions339
8.13 Hands on Lab340
8.13.1 JButton340
8.13.2 Action Event340
8.13.3 Dialogs340
8.13.4 Layout Managers341
8.13.5 Focus Listener341
8.13.6 Key Listener343
8.13.7 Mouse Listener344
8.13.8 MVC345
8.14 Exercises348
Chapter 9 Multithreading351
9.1 Processes and Threads351
9.2 Threads in Java354
9.3 Thread States357
9.4 Thread Scheduling and Priority359
9.5 Sharing Access to Data361
9.6 Synchronized Methods363
9.6.1 Motivation364
9.6.2 Stack with Synchronized Methods367
9.6.3 Producer and Consumer Problem368
9.7 Atomic Variables373
9.8 Executors377
9.9 Callable & Future380
9.10 BlockingQueue382
9.11 New Terminology385
9.12 New Words and Expressions386
9.13 Hands on Lab386
9.13.1 Unresponsive User Interface386
9.13.2 Thread Priority386
9.13.3 Unsynchronized Counter387
9.13.4 Volatile390
9.13.5 Synchronized Methods390
9.13.6 Atomic Variables390
9.13.7 Executor390
9.13.8 Blocking Queue391
9.14 Exercises394
Appendix Ⅰ Java Modifiers396
Appendix Ⅱ Java Documentation397
Appendix Ⅲ Unicode Chart(Basic Latin)402
Appendix Ⅳ Helpful Eclipse Shortcuts406
References408